Outline Standalone Outline For Mac
A view that uses a row-and-column format to display hierarchical data like directories and files that can be expanded and collapsed.
Framework
- AppKit
Outline Client. The Outline Client is a cross-platform VPN or proxy client for Windows, macOS, iOS, Android, and ChromeOS. The Outline Client is designed for use with the Outline Server software, but it is fully compatible with any Shadowsocks server. The client's user interface is implemented in Polymer 2.0. How to Add an Outline to a Google Doc on PC or Mac. This wikiHow teaches you how to add an outline to a Google Docs file. Outlines allow you to easily navigate longer documents by clicking headings in a list. Go to https://docs.google.com.
Declaration
Overview
Like a table view, an outline view does not store its own data, instead it retrieves data values as needed from a data source to which it has a weak reference (see Delegates and Data Sources). See NSOutlineViewDataSource
, which declares the methods that an NSOutlineView
object uses to access the contents of its data source object.
An outline view has the following features:
A user can expand and collapse rows, edit values, and resize and rearrange columns.
Each item in the outline view must be unique. In order for the collapsed state to remain consistent between reloads the item's pointer must remain the same and the item must maintain
isEqual(_:)
sameness.The view gets data from a data source (see
NSOutlineViewDataSource
).The view retrieves only the data that needs to be displayed.
Important
It is possible that your data source methods for populating the outline view may be called before awakeFromNib()
if the data source is specified in Interface Builder. You should defend against this by having the data source’s outlineView(_:numberOfChildrenOfItem:)
method return 0
for the number of items when the data source has not yet been configured. In awakeFromNib()
, when the data source is initialized you should always call reloadData()
.
For more information about using NSOutlineView in your app, see Navigating Hierarchical Data Using Outline and Split Views.
Subclassing
Subclassing NSOutlineView
is not recommended. Customization can be accomplished in your data source class implementation (conforming to NSOutlineViewDataSource
) or your delegate class implementation (conforming to NSOutlineViewDelegate
).
Topics
var dataSource: NSOutlineViewDataSource?
The object that provides the data displayed by the receiver.
var stronglyReferencesItems: Bool
A Boolean value that indicates whether the outline view retains and releases the objects returned from its data source.
func isExpandable(Any?) -> Bool
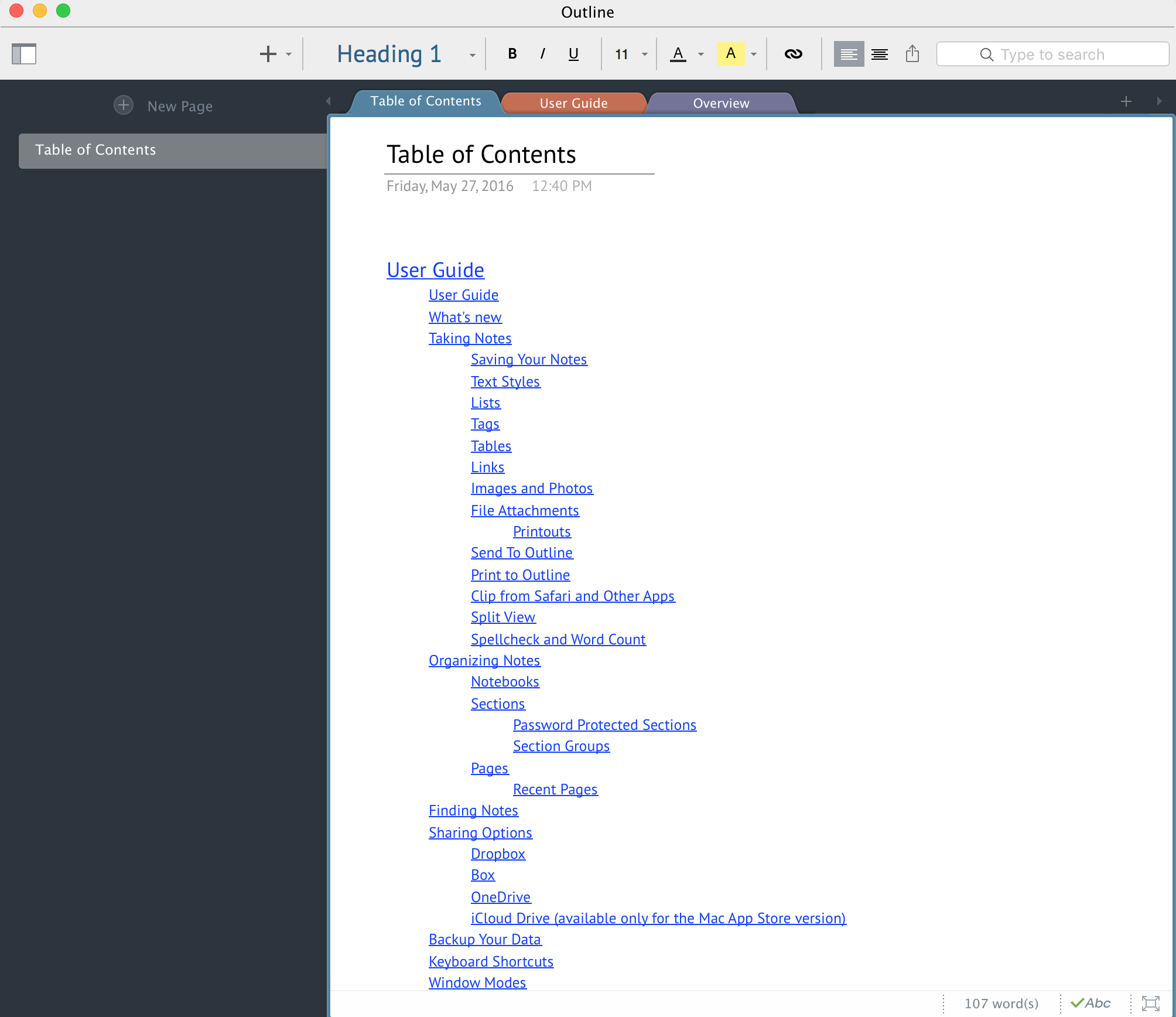
Returns a Boolean value that indicates whether a given item is expandable.
func isItemExpanded(Any?) -> Bool
Returns a Boolean value that indicates whether a given item is expanded.
func expandItem(Any?)
func expandItem(Any?, expandChildren: Bool)
Expands a specified item and, optionally, its children.
func collapseItem(Any?)
func collapseItem(Any?, collapseChildren: Bool)
Collapses a given item and, optionally, its children.
func reloadItem(Any?)
Reloads and redisplays the data for the given item.
func reloadItem(Any?, reloadChildren: Bool)
Reloads a given item and, optionally, its children.
func item(atRow: Int) -> Any?
func row(forItem: Any?) -> Int
var outlineTableColumn: NSTableColumn?

The table column in which hierarchical data is displayed.
var autoresizesOutlineColumn: Bool
A Boolean value that indicates whether the outline view resizes its outline column when the user expands or collapses items.
func level(forItem: Any?) -> Int
func level(forRow: Int) -> Int
var indentationPerLevel: CGFloat
var indentationMarkerFollowsCell: Bool
A Boolean value indicating whether the indentation marker symbol displayed in the outline column should be indented along with the cell contents.
To put a spinner control onto a worksheet in Excel 2011 for Mac, take these steps: While your form is unprotected, click the Spin Button control on the Developer tab of the Ribbon. Drag diagonally and then let go of the mouse. Right-click the new spinner control and choose Format Control from the. Created a UserForm (with ID: 'AssessmentForm') in MS-Excel for Mac 2011 using VBA and the VB Editor. Programmatically added eleven (11) 'SpinButtons' using this code sequence: For iLoop =. Spin Button 1. On the Developer tab, click Insert. In the ActiveX Controls group, click Spin Button. Drag a spin button on your worksheet. Right click the spin button (make sure Design Mode is selected). Click View Code. To link this spin button to a cell, add the following code. Excel for Windows & Mac - all versions. Posts 26,439. Re: Spin button suddenly stopped working Hi, Difficult to say without seeing the request in context but try changing the.value property to.listindex Richard Buttrey. Spin button suddenly stopped working. The Format Control option via the contextual menu is inadequate as the maximum allowable range is 30000 in it I have tried using a macro but both the 'up' & 'down' buttons on the Spin Button only increase the value. Any help would be appreciated! Excel scroll bar.
var autosaveExpandedItems: Bool
A Boolean value indicating whether the expanded items are automatically saved across launches of the app.
func setDropItem(Any?, dropChildIndex: Int)
func shouldCollapseAutoExpandedItems(forDeposited: Bool) -> Bool
Returns a Boolean value that indicates whether auto-expanded items should return to their original collapsed state.
func parent(forItem: Any?) -> Any?
func childIndex(forItem: Any) -> Int
Returns the child index of the specified item within its parent.
func child(Int, ofItem: Any?) -> Any?
func numberOfChildren(ofItem: Any?) -> Int
Returns the number of children for the specified parent item.
func frameOfOutlineCell(atRow: Int) -> NSRect
Returns the frame of the outline cell for a given row.
var delegate: NSOutlineViewDelegate?
func insertItems(at: IndexSet, inParent: Any?, withAnimation: NSTableView.AnimationOptions)
Inserts new items at the given indexes in the given parent with the specified optional animations.
func moveItem(at: Int, inParent: Any?, to: Int, inParent: Any?)
Moves an item at a given index in the given parent to a new index in a new parent.
func removeItems(at: IndexSet, inParent: Any?, withAnimation: NSTableView.AnimationOptions)
Removes items at the given indexes in the given parent with the specified optional animations.
var userInterfaceLayoutDirection: NSUserInterfaceLayoutDirection
This constant defines an index that allows you to drop an item directly on a target.
Outline View Button KeysThese keys are used by the outline view to create disclosure buttons that collapse and expand items.
class let columnDidMoveNotification: NSNotification.Name
Posted whenever a column is moved by user action in an NSOutlineView
object.
class let columnDidResizeNotification: NSNotification.Name
Posted whenever a column is resized in an NSOutlineView
object.
class let itemDidCollapseNotification: NSNotification.Name
Posted whenever an item is collapsed in an NSOutlineView
object.
class let itemDidExpandNotification: NSNotification.Name
Posted whenever an item is expanded in an NSOutlineView
object.
class let itemWillCollapseNotification: NSNotification.Name
Posted before an item is collapsed (after the user clicks the arrow but before the item is collapsed).
class let itemWillExpandNotification: NSNotification.Name
Posted before an item is expanded (after the user clicks the arrow but before the item is collapsed).
class let selectionDidChangeNotification: NSNotification.Name
Posted after the outline view's selection changes.
class let selectionIsChangingNotification: NSNotification.Name
Posted as the outline view’s selection changes (while the mouse button is still down).
Relationships
See Also
Navigating Hierarchical Data Using Outline and Split ViewsBuild a structured user interface that simplifies navigation in your app.
When working in Office 2011 for Mac, you’ll likely need to know how to create a multilevel numbered list in Word 2011. The easiest way is to start with a list that’s been indented with tabs. In a multilevel numbered list, a number denotes each new item in the list. Indents invoke formatting rules for sub-numbering.
Follow these steps to create multilevel numbered lists in Word 2011 for Mac:
Type a simple list.
Use the Tab key to indent the text in your list.
Select the list.
You can use any sequence of small paragraphs in a document.
On the Ribbon’s Home tab, go to the Paragraph group and click the Numbered List button (the middle of the three bullet and number buttons).
Word automatically senses you have a multilevel list and formats it.
To apply a new multilevel style, click anywhere in your list and choose the Multilevel List button. It’s the button at the right of the group of three bullet and numbering buttons on the Ribbon. Clicking the button displays the Multilevel List gallery. Choose a multilevel style from Current List, List Library, or Lists in Current Documents.
You can create your own multilevel list formats. Choosing the Define New Multilevel Listoption on the Multilevel style palette displays the Customize Outline Numbered List dialog, which lets you customize multilevel lists and outline styles by level.
From the Level list, choose which indentation level you want to format.
In the Number Format area, choose from the following options:
Enter a potential number candidate, such as a letter or number from your keyboard, in the text box.
Choose a style from the Number Style pop-up menu.
Use the Start At Spinner if you want the numbered list to start at a number other than 1 or letter a.
Select a level from the Previous Level Number pop-up menu to bring the number format from the chosen level to the level you’re formatting.
Click the Font button to display the Format Font dialog. Choosing this applies formatting to the level being formatted, not the Previous Level.
Under Number Position, you find these options:
Choose Left, Centered, or Right from the pop-up menu to change the alignment.
Use the Aligned At spinner control or type into the input box to set the number position alignment.
The Text Position area offers these goodies:
Select the Add Tab Stop At check box to add a tab stop at the position you specify using the spinner control.
Use the Indent At spinner control to set the bullet’s indentation.
Clicking the Show/Hide More Options button exposes more options.